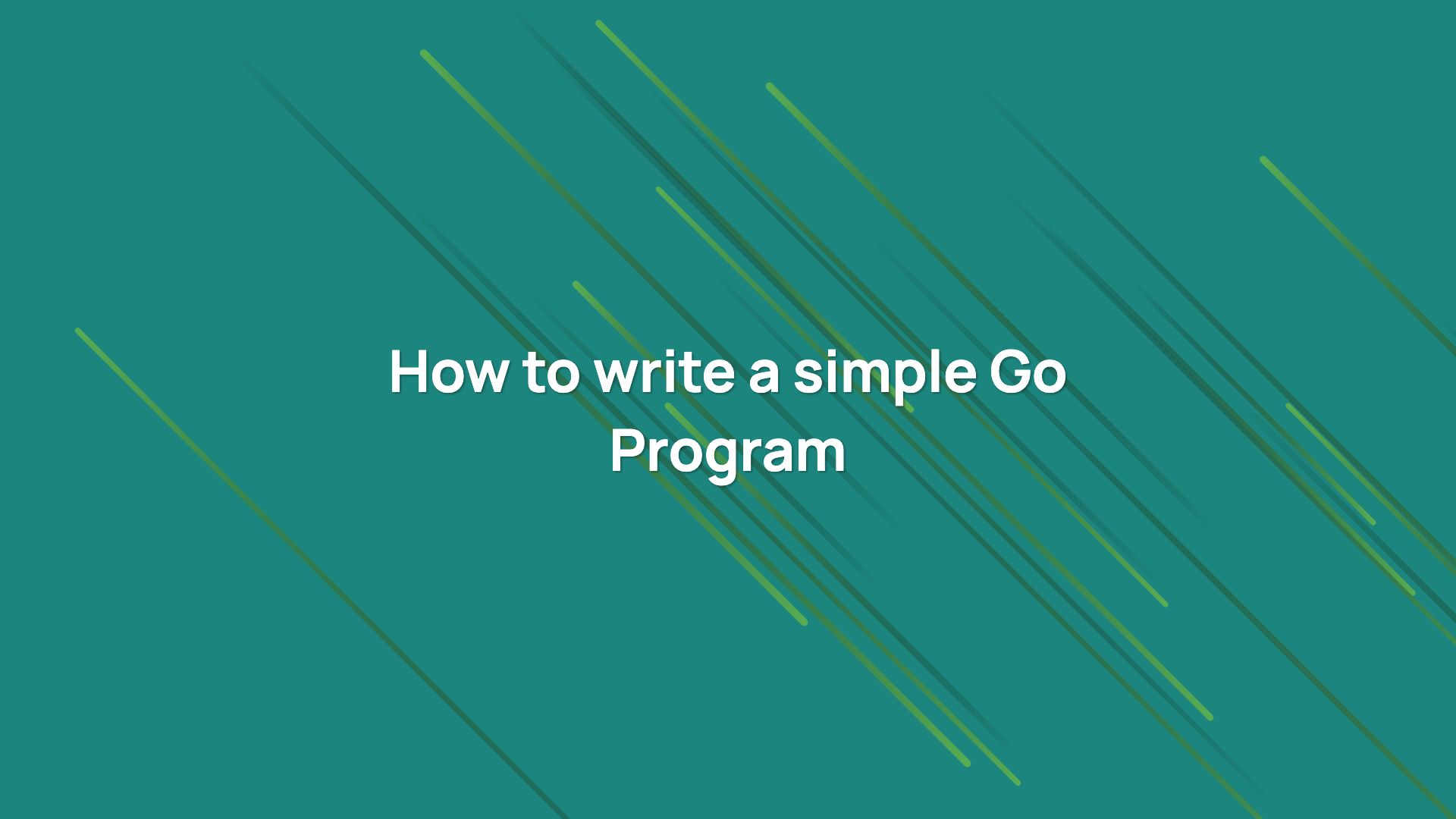
What is a Go program?
A Go program file is a simple UTF-8 text file with .go
extension. Hence you can edit a Go program in simple text editors like notepad, sublime text or IDEs like VSCode or WebStorm. You can use terminal editors like vim or nano too.
đź’ˇ If you are using VSCode, then install vscode-go extension. This will help you write better code and make formatting/debugging easier.
Go program structure
Every Go program must be included in a package. A standalone executable Go program must have a main
function and must be included in the main
package. The main
function is the entry point of the execution.
đź’ˇ A standalone executable program is a Go program that can be built to an executable using
go build
command or ran usinggo run
command.
package main
import "fmt"
func main() {
fmt.Println("Hello World!")
}
In the program above, we have defined the package main
at the top of the file because this is our standalone executable program and we also added main
method which will get executed when this program runs.
We also imported the fmt
package from Go’s standard library (it comes with Go installation). To import a package, we use the import
keyword followed by the name of the package in double-quotes.
The fmt
package is used here to print messages to the standard output. This package provides different functions to log any data to the standard output (STDOUT) in different formats.
In the above example, we have used Println
function which prints arguments as strings to the standard output or terminal.
Running a Go program
To run a Go program (assuming you have installed Go on your system), you need to instruct the Go compiler to compile and run a program using go run
command with the relative or absolute path of the Go program file.
If we put our earlier Go program code in hello.go
file, then the command to run this program from the same directory of this file would be as below.
$ go run hello.go
This will yield the following result in the terminal.
Hello World!
If you want to run multiple Go files at once, you can give a glob pattern or mention all the files in just one command
$ go run dir/*.go
$ go run dir/**/*.go
$ go run file-a.go file-b.go file-c.go
You can run multiple Go files at once because you might have separated different parts of your project code in their own separate files. This is helpful to manage large projects and make your code modular.
However, only one main
method can exist among them since there can be only one entry point of the execution and all program files should belong to the same directory (usually in the parent directory of the project).
If a file depends on a function or a variable that exists in another file, then you also need to add that file in the compilation phase using run
or build
command. This is exactly how we compile a C
or C++
project.
đź’ˇ This will be explained in further details in upcoming chapters.
To create a binary executable file, you can use the go build
command.
$ go build hello.go
The above command will create a binary executable file hello in the current directory which you can execute from terminal/command prompt.
$ ./hello
To deploy the binary files to bin
directory, you can use the below command.
$ go install hello.go
This will install hello
binary file in bin
directory of your current Go workspace (GOBIN
directory). Since, bin
directory is in the PATH
of your system, we can execute it from anywhere.
$ hello
Hello World!
If you are working with multiple files, the go install *.go
or go install file-1.go file-2.go ...
command will create a binary file in GOBIN
directory with a non-specific filename.
This filename is chosen from the filename of one of the files provided in the command that comes first in alphabetical order. However, this changes when we are compiling a standalone or distributable package. You will learn about this case in the packages lecture.
Comments
Like comments used in programming languages like JavaScript
or C++
, Go uses the same comment format. For single-line comment, you can use //comment
and for block comments, you can use /*comment*/
.
// I am a single line comment.
// I am another single line comment.
/*
I am a block comment.
And GoLang is awesome.
Say it with me, GoLang is awesome.
*/
Semicolons
Until now you might have noticed that we haven’t used semicolons in our programs which are heavily used in other programming languages like C
, C++
or JavaScript
.
Like C
, Go’s formal grammar uses semicolons to terminate statements, but unlike in C
, those semicolons do not appear in the source code. Instead Go’s Lexer program uses a simple rule to insert semicolons automatically as it scans your Go program, so the source code is mostly free of them.
The rule is this. If the last token before a newline is an identifier (which includes words like int
and float64
), a basic literal such as a number
or string
constant, or one of the below tokens
break continue fallthrough return ++ -- ) }
then Lexer always inserts a semicolon after this token. This could be summarized as, “if the newline comes after a token that could end a statement, insert a semicolon”.
Hence, Go code is free of semicolons. If you accidentally write semicolons in the source code, VSCode plugin will strip them off on save. You can also use gofmt
command to format your code as per the Go recommendations.
The only place you will find semicolons is where statements have to be terminated deliberately using ;
(semicolon) like for
,switch
, if
statements or other variations of them.
You are free to use semicolons whenever you want, but that won’t be idiomatic Go code. For example, you could write the following code and the program will still execute.
package main
import("fmt"; "math";);
func main() {
fmt.Println(math.Sqrt(16));
};
But the following syntax is the idiomatic way to write Go code.
package main
import(
"fmt" // ;
"math" // ;
) // ;
func main() {
fmt.Println(math.Sqrt(16)) // ;
} // ;
Best practices
Go is a very clean and systematic language. Hence your code must follow community guidelines and Go specifications. gofmt
is a tool that automatically formats Go source code, which can be helpful to write Go idiomatically.
Effective Go
Follow the Effective Go documentation to understand these standard practices. https://golang.org/doc/effective_go.html
Go Playground
Go community has created an online IDE to play with simple Go programs. This is like JSFiddle or CodePen but strictly for Go Programing language.
I would recommend to not use this IDE every single time because it is hosted on powerful servers. In my experience, I always get different experiences and results on the local system compared to this IDE, like for example, while testing concurrency related programs and heavy networking and I/O operations. But for other simple programs, feel free to use it.