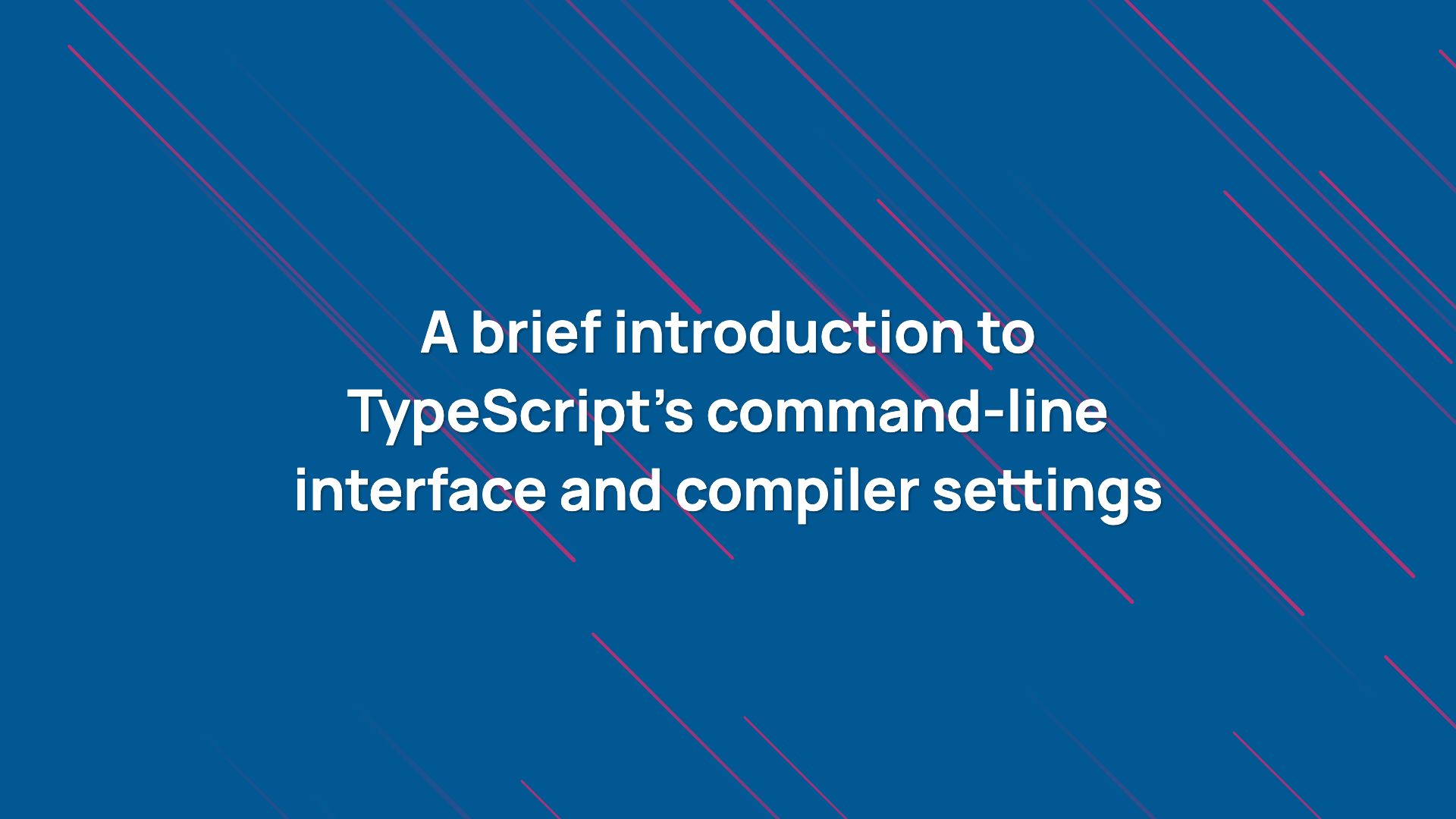
In the previous TypeScript Compilation lesson, we learn about different TypeScript compiler settings and the role of the tsconfig.json
file. Also, we learned how to best optimize the TypeScript compiler as per our needs. These settings can be controlled using the command-line options as well which we will learn in this lesson.
The tsc
command envokes the TypeScript compiler. When no command-line options are present, this command looks for the tsconfig.json
file. If no tsconfig.json
is found, it returns by dumping the usage of tsc
command.
The minimal thing needed by the tsc
command is the location of input source files to compile. We can provide these using files
or include
options of the tsconfig.json.
However, we can also provide these files directly through the command itself.
$ tsc a.ts src/**/*.ts
The command above compiles the a.ts
and all the .ts
files inside ./src
directory using the default compiler-options values. This is equivalent of having a tsconfig.json
files with include
option containing these exact paths. Since no ourDir
option is involved in the compilation, the ouput .js
files are places besides .ts
files.
When the source files are provided in the command-line itself like the above, the tsconfig.json
file is ignored even if it is present in the project. Therefore, all the compiler options need to be provided via command itself.
π‘ The reverse is not true. You can provide extra compiler-options or override exisiting ones via command if you are using
tsconfig.json
file.
Compiler-Option Flags
These flags control the value of compile-options we saw earlier in the TypeScript Compilation lesson. These names of these flags are similar to the compiler-option names used in the tsconfig.json
.
π‘ You can drop the double-quotes while specifying a flag value. But I would recommed you to use double-quote (
"value"
) to avoid unnecessary side effects.
I have mentioned the compiler-option name used inside tsconfig.json
in the parentheses below. Therefore, I would recommend visiting the Compilation lesson since I have explained these options there.
β --outDir
(outDir)
Sets the output directory path to in which the compilation files such as .js
, .map
and .d.ts
, etc. needs to be generated.
$ tsc --outDir "./dist"
β --rootDir
(rootDir)
Sets the root directory path (common longest path among input source files) to produce the desired output file tree structure.
$ tsc --rootDir ".."
β --removeComments
( removeComments)
Tells the TypeScript compiler to remove the JavaScript comments from the output JavaScript code.
$ tsc --removeComments
β --module
or -m (module)
Sets the desired module system while producing compiled JavaScript code.
$ tsc -m "CommonJS"
$ tsc --module "CommonJS"
β --outFile
(outFile)
Sets the output file path while producing a JavaScript bundle.
tsc --outFile "./dist/bundle.js"
β --sourceMap
(sourceMap)
Tells** the TypeScript compiler to produce a .map
file (source-map) for every input source file (.ts
or .js
).
$ tsc --sourceMap
β --mapRoot
(mapRoot)
Sets the root for the source-map URLs if the --sourcemap
flag is also provided.
$ tsc --sourcemap --mapRoot "http://debug.com/dist"
β --inlineSourceMap
(inlineSourceMap)
Tells the TypeScript compiler to generate inline source-maps in the output JavaScript files.
$ tsc --inlineSourceMap
β --sourceRoot
(sourceRoot)
Sets the root of source files in the source-maps whether source-maps are inline or external files.
$ tsc --sourceMap --sourceRoot "http://source.com/"
$ tsc --inlineSourceMap --sourceRoot "http://source.com/"
β --inlineSources
(inlineSources)
Tells the TypeScript compiler to inline sources in the source-maps whether source-maps are inline or external files.
$ tsc --sourceMap --inlineSources
$ tsc --inlineSourceMap --inlineSources
β --declaration
or -d
(declaration)
Tells the TypeScript compiler to produce type declaration files (.d.ts).
$ tsc -d
$ tsc --declaration
β --declarationDir
(declarationDir)
Sets the output directory to place declaration files if --declaration
flag is provided.
$ tsc --declaration --declarationDir "./dist/types"
β --declarationMap
(declarationMap)
Tells the TypeScript compiler to produce .d.ts.map
(source-maps for the type declaration .d.ts
files) files when --declaration
flag is provided.
$ tsc --declaration --declarationMap
β --lib
(lib)
Tells the TypeScript compiler to import type declarations from the TypeScript standard library (provided by the installation).
$ tsc --lib "DOM,ES2015"
β --noLib
(noLib)
Tells the TypeScript compiler to not to import any type declarations from the TypeScript standard library
$ tsc --noLib
β --typeRoots
(typeRoots)
Sets the directories from which all the type declaration packages need to be imported implicitly.
$ tsc --typeRoots "./my-types,./vendor"
β --types
(types)
Tells the TypeScript compiler to selectively import the given type declaration packages from the type roots.
$ tsc --types "node,moment"
β --allowJs
(allowJs)
Tells the TypeScript compiler to consider files ending with .js
(JavaScript files) to include in the compilation.
$ tsc --allowJs
β --checkJs
(checkJs)
Tells the TypeScript compiler to consider files ending with .js
(JavaScript files) for static type analysis.
$ tsc --checkJs
β --target
or -t
(target)
Sets the compilation target using which the TypeScript compiler optimizes output JavaScript code and sets the default value for lib
compiler-option.
$ tsc -t "ES5"
$ tsc --target "ES5"
β --downlevelIteration
(downlevelIteration)
Tells the TypeScript compiler to down compile iterations (such as for-of loop, spread operator, etc.) when the target
doesnβt support it.
$ tsc --downlevelIteration
β --importHelpers
(importHelpers)
Tells the TypeScript compiler to **add tslib
import statements** for the helper function used in the down compiled code.
$ tsc --importHelpers
β --moduleResolution
(moduleResolution)
Tells the TypeScript compiler to use a specific module resolution strategy while looking for the module files (of the import statements).γdeprecatedγ
$ tsc --moduleResolution "Node"
β --resolveJsonModule
(resolveJsonModule)
Tells the TypeScript compiler to also look for .json
file while resolving a module.
$ tsc --resolveJsonModule
β --esModuleInterop
(esModuleInterop)
Tells the TypeScript compiler to generate helper function in the compiled JavaScript code to increase the interoperability between CommonJS and ECMAScript modules.
$ tsc --esModuleInterop
β --allowSyntheticDefaultImports
(allowSyntheticDefaultImports)
Instructs the TypeScript compiler to allow default import from a module that doesnβt have an explicit default export.
$ tsc --allowSyntheticDefaultImports
β --baseUrl
(baseUrl)
Sets a common directory path from which all non-relative module imports should be first searched before searching inside node_modules
directory.
$ tsc --baseUrl "."
β --strict
(strict)
Instructs the TypeScript compiler to enable all strict family options (mentioned below).
$ tsc --strict
β --alwaysStrict
(alwaysStrict)
_Tells the TypeScript compiler to add _*"use strict";*
* annotation in the compiled JavaScript code to enable *ES 5 Strict Mode.
$ tsc --alwaysStrict
β --noImplicitAny
(noImplicitAny)
Tells the TypeScript compiler to throw an error when an entity has the any
type which was provided by the TypeScript implicitly (by default).
$ tsc --noImplicitAny
β --noUnusedParameters
(noUnusedParameters)
Tells the TypeScript compiler to throw an error when function parameters were defined but not used in the function definition.
$ tsc --noUnusedParameters
β --noUnusedLocals
(noUnusedLocals)
Tells the TypeScript compiler to throw an error when local variables (or constants) were defined but never used.
$ tsc --noUnusedLocals
β --noImplicitReturns
(noImplicitReturns)
Tells the TypeScript compiler to throw an error when a function has a return type but return value was not provided in some logical scenarios.
$ tsc --noImplicitReturns
β --noFallthroughCasesInSwitch
(noFallthroughCasesInSwitch)
Tells the TypeScript compiler to throw an error when a case
block inside a switch
statement doesnβt use return
or break
statement.
$ tsc βnoFallthroughCasesInSwitch
β --noImplicitThis
(noImplicitThis)
Tells the TypeScript compiler to throw an error when this
is not known.
$ tsc --noImplicitThis
β --strictNullChecks
(strictNullChecks)
Tells the TypeScript compiler to throw an error when an entity of concrete type such as string
, number
, etc. is assigned with a null
or undefined
value.
$ tsc --strictNullChecks
β --listFiles
(listFiles)
Tells the TypeScript compiler to display input source file paths (such as .ts
, .d.ts
, .js
, etc.) at the end of the compilation.
$ tsc --listFiles
β --listEmittedFiles
(listEmittedFiles)
Tells the TypeScript compiler to display output compilation file paths (such as .js
, .d.ts
, .map
, etc.) at the end of the compilation.
$ tsc --listEmittedFiles
β --noEmit
(noEmit)
Tells the TypeScript compiler to prevent outputting any output compilation file paths (such as .js
, .d.ts
, .map
, etc.).
$ tsc --noEmit
β --noEmitHelpers
(noEmitHelpers)
Tells the TypeScript compiler to prevent adding any helper functions (or their import statements) in the compiled JavaScript code (while down compiling the code).
$ tsc --noEmitHelpers
β --noEmitOnError
(noEmitOnError)
Tells the TypeScript compiler to prevent outputting any output compilation file paths (such as .js
, .d.ts
, .map
, etc.) when there are compilation errors.
$ tsc --noEmitOnError
β --emitDeclarationOnly
(emitDeclarationOnly)
Tells the TypeScript compiler to emit only declaration files (.d.ts
).
$ tsc --emitDeclarationOnly
Tooling Flags
β --init
Creates an empty TypeScript project with a sample tsconfig.json
file.
$ tsc --init
β --forceConsistentCasingInFileNames
Tells the TypeScript compiler to throw an error when multiple import statements of the same module have different letter casing.
$ tsc --forceConsistentCasingInFileNames
β --project <path>
or -p <path>
Point to a custom tsconfig.json
file-path instead of using the default one.
$ tsc --project ./configs/tsconfig.prod.json
β --watch
or -w
Run TypeScript compiler in watch mode. This flag will keep the TypeScript compiler running in the background watching for any file changes included in the compilation.
$ tsc --watch
β --showConfig
Show configuration settings interpreted by the TypeScript compiler.
$ tsc --showConfig
β --diagnostics
and --extendedDiagnostics
These flags display diagnostics information of the compilation process.
$ tsc --diagnostics
$ tsc --extendedDiagnostics
Files: 17
Lines: 27018
Nodes: 118393
Identifiers: 43892
Symbols: 30151
Types: 9399
Instantiations: 5009
Memory used: 68974K
I/O read: 0.01s
I/O write: 0.00s
Parse time: 0.33s
Bind time: 0.19s
Check time: 0.93s
Emit time: 0.02s
Total time: 1.47s
β --version
or -v
Show the TypeScript version.
$ tsc -v
Version 3.9.3
β --help
or -h
Show tsc
command usage information.
TypeScript comes with many useful compiler-options that we might not have covered in this lesson. Use this documentation to look for these.